Idiom #27 Create a 3-dimensional array
Declare and initialize a 3D array x, having dimensions boundaries m, n, p, and containing real numbers.
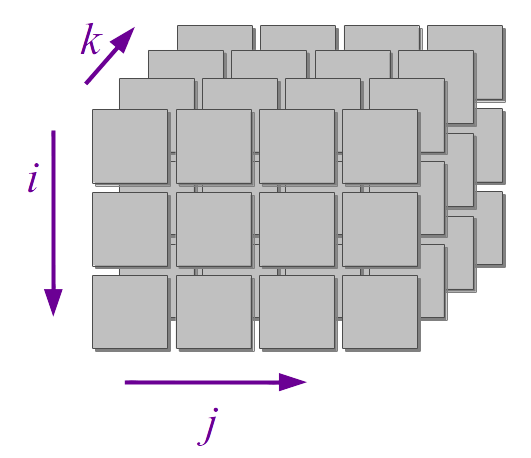
- Ada
- C
- C++
- D
- Dart
- Elixir
- Erlang
- Fortran
- Go
- Go
- Go
- Haskell
- JS
- Java
- Java
- Kotlin
- Lisp
- Lua
- Obj-C
- PHP
- Pascal
- Perl
- Perl
- Python
- Python
- Python
- Python
- Ruby
- Rust
- Rust
var x = new List.generate(m, (_) =>
new List.generate(n, (_) =>
new List.filled(p, 0.0),
growable: false),
growable: false);
Dart does not have multidimensional arrays as a primitive, this is a list of lists of lists of doubles, all fixed-length lists.
def main(m, n, p) do
if m == 0 or n == 0 or p == 0 do
[]
else
for _ <- 1..m, do: for _ <- 1..n, do: for _ <- 1..p, do: 0
end
end
X = array(M, N, P).
-spec array(pos_integer(), pos_integer(), pos_integer()) -> [[[float()]]].
array(M, N, P) -> [array(M, N) || _ <- lists:seq(1, P)].
array(M, N) -> [array(M) || _ <- lists:seq(1, N)].
array(M) -> [rand:uniform() || _ <- lists:seq(1, M)].
func make3D(m, n, p int) [][][]float64 {
buf := make([]float64, m*n*p)
x := make([][][]float64, m)
for i := range x {
x[i] = make([][]float64, n)
for j := range x[i] {
x[i][j] = buf[:p:p]
buf = buf[p:]
}
}
return x
}
This works even when m, n, p are not compile-time constants.
This code allocates one big slice for the numbers, then a few slices for intermediate dimensions.
To same function would be rewritten, for types other than float64.
This code allocates one big slice for the numbers, then a few slices for intermediate dimensions.
To same function would be rewritten, for types other than float64.
const m, n, p = 2, 2, 3
var x [m][n][p]float64
m, n, p must be constant for this syntax to be valid.
Here x is of type [2][2][3]float64, it is not a slice.
Here x is of type [2][2][3]float64, it is not a slice.
func make3D[T any](m, n, p int) [][][]T {
buf := make([]T, m*n*p)
x := make([][][]T, m)
for i := range x {
x[i] = make([][]T, n)
for j := range x[i] {
x[i][j] = buf[:p:p]
buf = buf[p:]
}
}
return x
}
This generic func works for any type parameter T.
m, n, p do not need to be compile-time constants.
This code allocates one big slice for the elements, then a few slices for intermediate dimensions.
m, n, p do not need to be compile-time constants.
This code allocates one big slice for the elements, then a few slices for intermediate dimensions.
double[][][] x = new double[m][n][p];
Initial values are 0.0
m, n, p need not be known at compile time
m, n, p need not be known at compile time
NSArray *x=@[
@[
@[@0.1, @0.2, ... ], // p column values
... // n sub-rows
],
... // m rows
];
Object arrays are one-dimensional, but can contain nested arrays (accessible later, if needed, through x[i][j][k] all right). With plain-C types it would be precisely same as in plain C. Compare also idiom #26
my $array3d = [
[ [ 1, 0, 1 ],
[ 0, 0, 0 ],
[ 1, 0, 1 ] ],
[ [ 0, 0, 0 ],
[ 0, 2, 0 ],
[ 0, 0, 0 ] ],
[ [ 3, 0, 3, ],
[ 0, 0, 0, ],
[ 3, 0, 3, ] ]
];
Array variables are only one-dimensional, but arrays-of-arrays using array references can represent multi-dimensional arrays.
my @x;
my ($m, $n, $p) = (4,3,2);
my $v = 0;
foreach my $mx (0..$m-1) {
foreach my $nx (0..$n-1) {
foreach my $px (0..$p-1) {
$x[$mx][$nx][$px] = $v++;
}
}
}
Sample boundaries are 4, 3, 2 and filled with integers. Perl will "autovivify" lists (i.e. add new elements) as they are referenced and grow the arrays on the fly.
x = numpy.zeros((m,n,p))
NumPy is a third-party library for scientific computing.
programming-idioms.org