This language bar is your friend. Select your favorite languages!
Select your favorite languages :
- Or search :
Idiom #169 String length
Assign to the integer n the number of characters of the string s.
Make sure that multibyte characters are properly handled.
n can be different from the number of bytes of s.
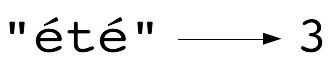
- Ada
- Clojure
- Clojure
- Cobol
- C++
- C#
- D
- D
- D
- Dart
- Elixir
- Erlang
- Fortran
- Go
- Haskell
- JS
- JS
- Java
- Kotlin
- Lisp
- Lisp
- Lua
- Lua
- Obj-C
- PHP
- Pascal
- Pascal
- Pascal
- Perl
- Python
- Ruby
- Ruby
- Rust
- Scala
- Smalltalk
- VB
(def n (.codePointCount s 0 (count s)))
This properly handles Unicode characters. Using count alone will not work for Unicode characters encoded in more than one byte.
auto utf8len(std::string_view const& s) -> size_t
{
std::setlocale(LC_ALL, "en_US.utf8");
auto n = size_t{};
auto size = size_t{};
auto mb = std::mbstate_t{};
while(size < s.length())
{
size += mbrlen(s.data() + size, s.length() - size, &mb);
n += 1;
}
return n;
}
N = string:length(S)
length(String::unicode:chardata()) -> integer() >= 0
Returns the number of grapheme clusters in String.
Returns the number of grapheme clusters in String.
int n = s.length();
This works only if s != null.
If s == null, this throws a NullPointerException.
If s == null, this throws a NullPointerException.
local utf8={}
function utf8.bytes(str,index)
local byte=string.byte(str,index)
local ret
if byte==nil then ret=0
elseif byte<128 then ret=1
elseif byte<192 then ret=2
elseif byte<224 then ret=3
else ret=4
end
return ret
end
function utf8.len(str)
local count=0
local fini=#str+1
local index=1
while index~=fini do
count=count+1
index=index+utf8.bytes(str,index)
end
return count
end
local n=utf8.len(s)
Use for lua version under 5.3. (which doesn't have utf8 lib)
n := s.length;
Works correctly if s is of type UnicodeString and the string is not encoded using combining characters.
n = len(s)
If s is a Python 3 str, len(s) returns the number of characters. If s is a Python 3 bytes, len(s) returns the number of bytes.